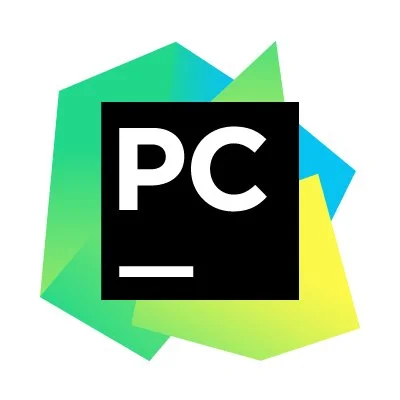
The Best PyCharm MayaPy Interpreter Setup
There’s no denying – Maya makes development hard.
Python is one of the most popular programming languages in the world as of 2022. The language supports many great workflows, but most are off-limits when working with Maya. Even just setting up an IDE with MayaPy has been a challenge through the years.
Today I will share how I set up PyCharm to work with MayaPy and how it enables a super-slick workflow for unit testing.
Getting Maya Python stub files
To get started, we’ll fetch Maya’s devkit. If you’re unfamiliar, the devkit contains autocomplete stubs for Maya’s Python modules. Download the appropriate one for your version of Maya from here.
With it downloaded, you’ll find the stub files at the following location inside: devkit/other/Python(x)/pymel/completion
There is a special kind of stub file PyCharm supports that we will take advantage of – pyi files! I reached out to Autodesk several years ago when I first learned of pyi files and asked them to begin including them with Maya distributions. So, if you see a folder named “pyi” in the devkit location from above, use it. If you are on a version of Maya where the devkit doesn’t include pyi files, we can make them ourselves! All you need to do is rename the extension of the .py Maya stubs to .pyi. Here is a short script to help you with the renaming.
import os
import shutil
def rename_from_py_to_pyi(py_dir, output_dir):
shutil.copytree(py_dir, output_dir)
for root, _, files in os.walk(output_dir):
py_files = [os.path.join(root, f) for f in files if f.endswith('.py')]
for f in py_files:
os.rename(f, '{}i'.format(f))
if __name__ == '__main__':
# Update below with the appropriate local paths for your machine
rename_from_py_to_pyi(r'/Users/mat/py', r'/Users/mat/pyi')
Find a nice home for these pyi stubs on your hard drive, as we will be using them when we set up our MayaPy interpreter. The most obvious place to put them is in the, otherwise empty, devkit folder in your Maya installation location.
Setting up the MayaPy interpreter
Now that you have a stubs folder full of .pyi files, it is time we set up our MayaPy interpreter in PyCharm. If you’ve done this before using past approaches, this time will be slightly different because of pyi, so be sure to follow along.
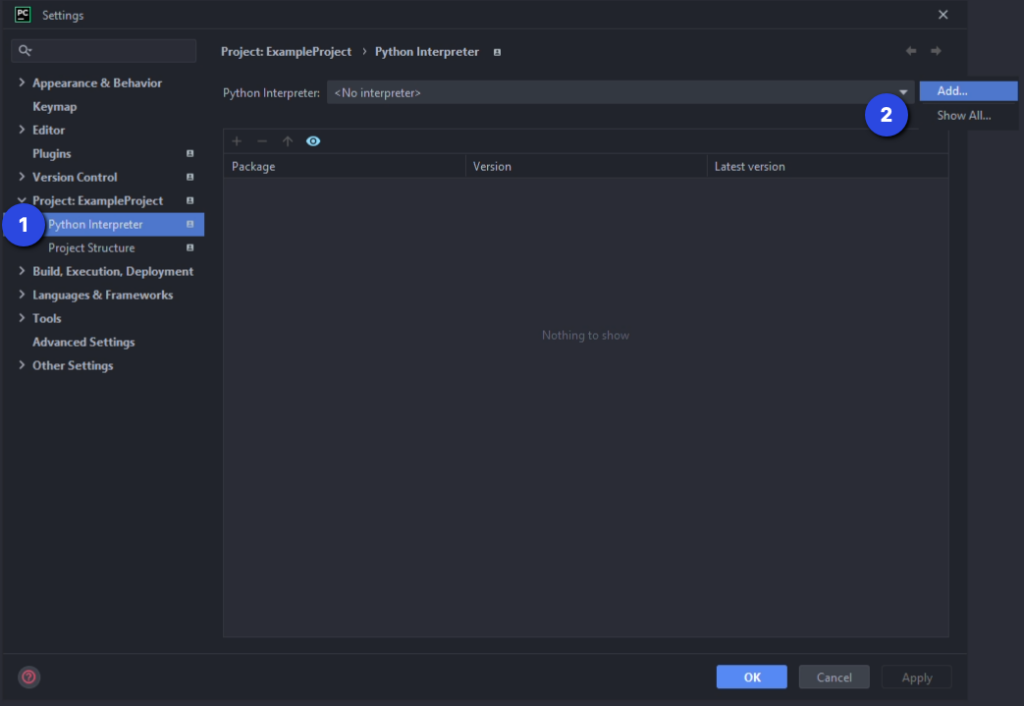
- In PyCharm’s Settings, go into “Project: <Your Project Name>” and choose “Python Interpreter” from the left-side menu.
- Click on the “…” button to the right of the “Python Interpreter:” dropdown and choose “Add…”
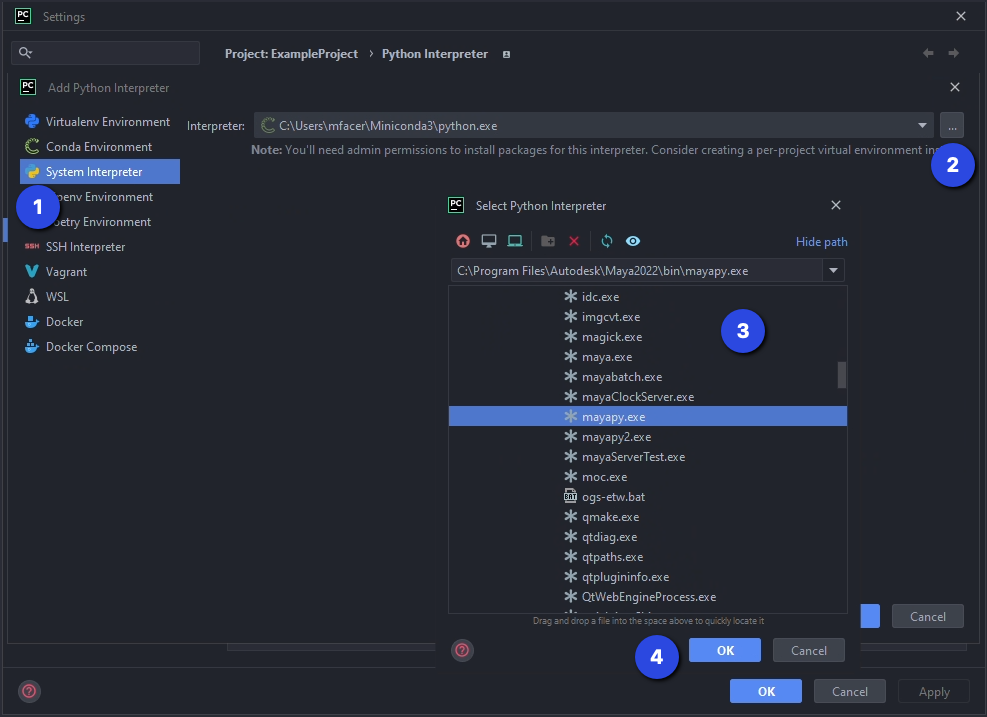
- Select “System Interpreter”.
- Press the “…” button to the right of the “Interpreter:” dropdown. It will pop up a new window.
- Navigate to your Maya installation’s bin folder and choose “mayapy.exe” (or “mayapy2.exe”).
- Press OK
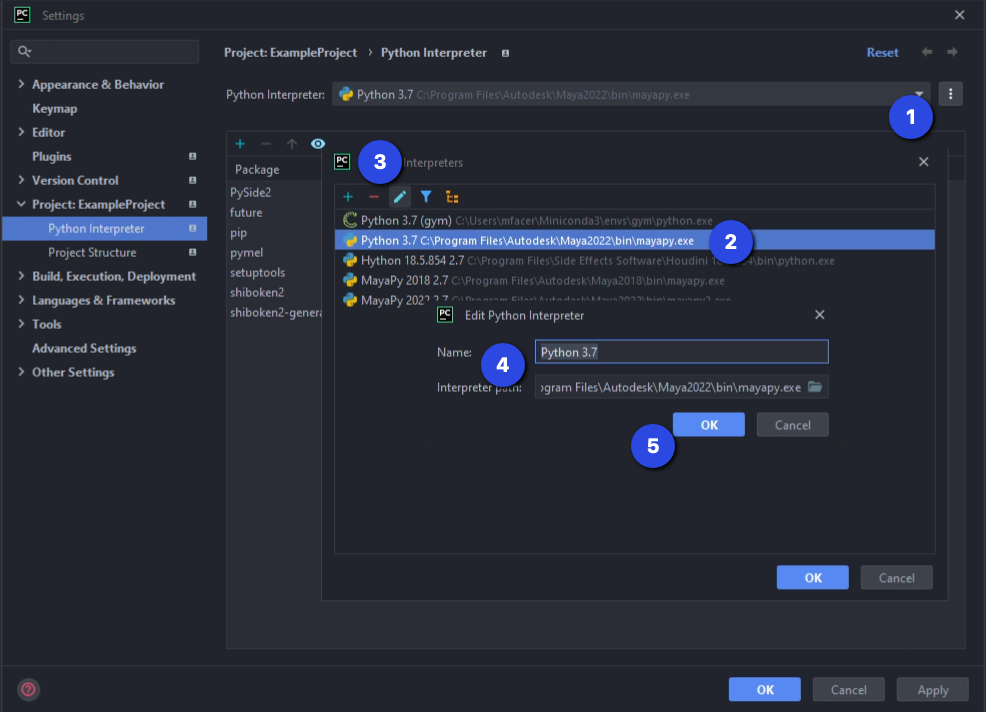
- Press the “…” button to the right of the “Python Interpreter:” dropdown again. This time choose “Show All…”
- From the list of interpreters that appears, choose the one you just made.
- Press the pencil, “Edit”, button to give it a better name.
- Rename it to something more descriptive. I like to follow the template of “ExeName AppVersion PythonVersion”, i.e. “MayaPy 2022 3.7”
- Press OK to accept your changes.
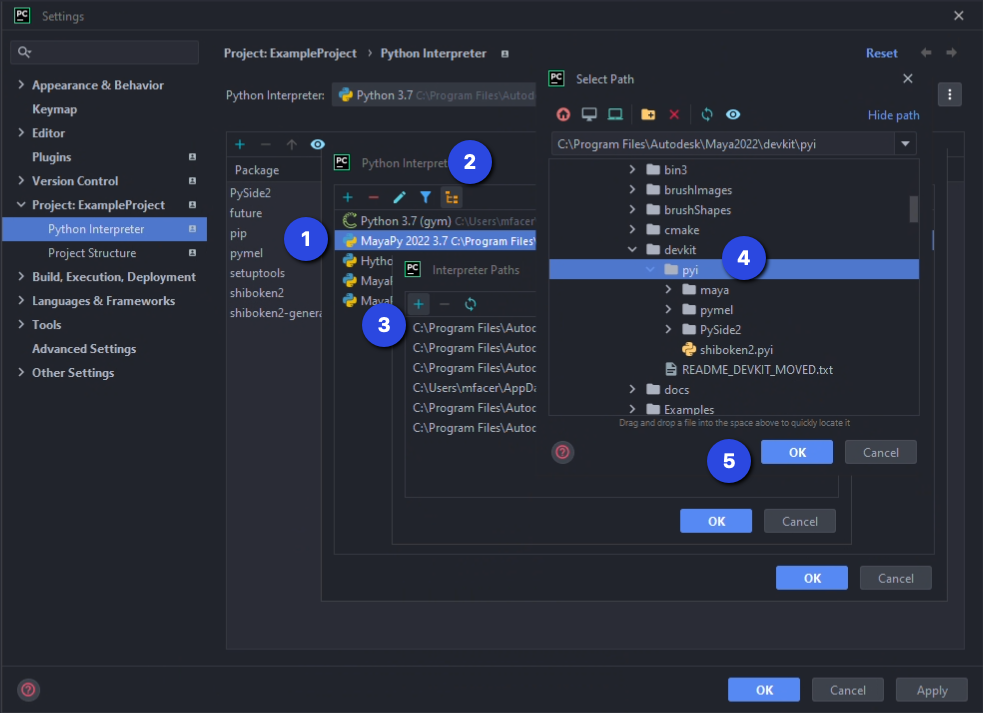
- Select your newly renamed Python Interpreter again from the previous window.
- This time, press the tree, “Show paths for the selected interpreter”, button.
- Press the “+” button to add a new path.
- Navigate to the “pyi” stubs folder that we made previously, wherever you decided to keep it.
- Press “OK” on all windows until your settings updates have been accepted.
You’re all done! Congrats!
There were a lot of steps here, but this is extremely straightforward after you’ve done it once.
Why is this awesome?
Direct Set-Up
This first reason is admittedly minor, but it is more direct than popular recommendations in the past. Previously it was suggested to do all of the steps above, but then also remove Maya’s site-packages folder from the interpreter paths to set up auto-complete properly. This removal step was always unintuitive but necessary so as to avoid conflicts between the stub files and the same modules found in Maya’s site-packages folder.
Why don’t we need to solve the conflict problem here? More on that in a bit.
All-encompassing Auto-complete
Because we do not remove Maya’s site-packages folder, if there are other packages or modules installed there you will get auto-complete for them also since they are all still on the pythonpath.
The Power of pyi
Coming back to the question, “why don’t we need to solve the conflict problem here?” The answer to that question comes from those pyi stubs we made.
The pyi files unlock special functionality with PyCharm. PyCharm knows to use these files in the editor for auto-complete purposes but then ignore them when running code and use the real modules instead. This is game-changing for MayaPy developers, and this is what makes this setup so great.
Why, you ask? Well, this magic brings our environment more in line with a regular Python development environment. You still can’t create virtual environments (at least I haven’t got that working yet), but we do unlock several other great workflows – and with minimal effort!
Unit testing
You can now take advantage of unit testing directly inside PyCharm! This works using the default built-in unittest module and with PyCharm’s test running interface.
The only thing you need to do to get this working? Initialize Maya! In your tests folder, create an __init__.py file and put your code there for initializing Maya’s standalone mode. Run your tests, and that __init__.py file will be run, and the rest just works.
Coverage
If you don’t already know, pip works with Maya and has for years. You can pip install the coverage package and run your unit tests with coverage now, too! PyCharm will give you line-by-line highlighting to show you what is covered and what is not.
__name__ == “__main__”
Similarly, slap that same maya.standalone.initialize()
code in a __name__ == “__main__”
block at the bottom of a file, followed by some test code for testing your module, and be able to run it. Super fast iteration without even having to launch Maya!
PySide iteration
Speaking of which, if you’re clever about structuring your PySide GUI classes, you can do most (if not all) of your GUI work by launching it from PyCharm now!
Create the main class to contain the bulk of your GUI, but omit code that handles integrating it with Maya’s GUI. Save that to be implemented in a Maya-specific subclass. Call the main class from within PyCharm, within your __name__ == “__main__”
block, and then call the Maya-specific class inside Maya. Say goodbye to all that unproductive time waiting for Maya to start up and shut down!
In closing
I’ve been using this PyCharm setup for several years, and I’ve been thrilled with it. It feels like a massive step toward normal Python development for Maya. Unit testing and improved iteration truly transform the experience. I hope others will be excited to discover how easy it is to unlock these features, and if you discover any other cool workflow improvements I’d love to hear about them!
Hello Mat, thanks for the great post. I am assuming at the time you wrote the post. You used an older version of PyCharm. As with the version, I started that endeavor, I am using PyCharm 2022.3.2, and I cannot replicate the last step. Do you have any tips that might help? Thanks
Hi Stephan, thanks for the comment and I’m glad you like the post. Can you specify which step you’re having difficulty with? I’d be happy to clarify (and update the post if needed!)
Hey, I downloaded the devKit from
https://aps.autodesk.com/developer/overview/maya
for maya 2023 and 2024 I cound not pyi folder at all.
Is it the wrong devKit or there is another way to make the autocompletion
The autocomplete stubs were generated via PyMel, which Autodesk doesn’t maintain. They’ve decided to no longer distribute PyMel after switching Maya to exclusively Python 3. We asked them about this and their guidance was to continue using the stubs from the 2022 devkit for Maya 2023 and 2024.
Not ideal, but it does the job still. I’ve noticed some Maya stubs projects starting to show up on GitHub, so that could be worth looking further into as well. The one that I tried pip installing was nested under a package named maya-stubs, making it so it didn’t work immediately. I just needed to rename it to maya, if I remember correctly.
Hi Matt, how come I can’t use that interpreter in different projects? If I want to I have to recreate it each time by pointing to the same .exe but it’s always missing the extra dependencies.
Hi Loic!
That sounds odd. Do you have a lot of interpreters in your PyCharm? The only time I remember having trouble finding them was when I had a lot of interpreters and they got pushed out from the drop down. I think there was an option to view more interpreters, where I found them.
Hi! Thanks a lot for this article, it got me almost there. My question is – is there a way for the interpreter to also autocomplete methods on named variables? Or did I setup something wrong?
For example
s = polySphere()[0] #polySphere autocompletes just fine
s. #nt.Transform s gives me nothing on autocomplete :C
This isn’t possible, unfortunately. Maya’s commands can return a variety of types from a single command, depending on the combination of flags you give as input.
If using Maya’s script editor to play around, and you have auto complete enabled in there, you’ll notice you’ll have auto complete in this situation if you’ve already run the previous line. Running the previous line lets the interpreter know what value ends up in the variable. Without running the previous line, you’ll notice the autocomplete doesn’t work.
There are ways to instruct PyCharm what value type you expect to be in the value, which will then manually enable autocomplete for that type. I’ll need to look it up, as it’s been a while since I’ve needed to.
Hey! Just had a moment to look it up.
You can add
# type:
to the end of your line where you’re creating your new variable, so# type: list
for exampleAlternatively, you can use Python3’s typing syntax and do
my_var: list = "this is a string, but will be treated as a list for auto-complete because I am forcing it"